In object-oriented software development, adhering to design principles is essential for building scalable, maintainable, and future-proof code. One such crucial principle is the Open/Closed Principle (OCP). This principle encourages developers to design classes and modules in a way that allows extension without modifying the existing code. In this article, we’ll explore the Open/Closed Principle using a real-world PHP 8 example and demonstrate how to apply it alongside PSR (PHP Standard Recommendation) best practices.
The Open/Closed Principle, part of the SOLID principles, suggests that classes should be open for extension but closed for modification. In other words, when new features or functionalities need to be added, we should extend the existing classes rather than modifying them directly. This helps maintain the stability of the existing codebase and reduces the chances of introducing bugs.
Let’s consider an example of a Payment Gateway System that processes payments for an e-commerce platform. We’ll start with a simple PaymentProcessor class that handles the payment processing for various payment methods, such as Credit Card and PayPal.
<?php
// PaymentProcessor.php
class PaymentProcessor
{
public function processPayment($paymentMethod, $amount)
{
if ($paymentMethod === 'CreditCard') {
// Logic to process credit card payment
} elseif ($paymentMethod === 'PayPal') {
// Logic to process PayPal payment
} else {
throw new \InvalidArgumentException('Invalid payment method');
}
}
}
The above implementation might work well initially, but it violates the Open/Closed Principle. Suppose we want to add a new payment method, ‘Stripe’, to our system. Modifying the existing class directly would break the principle.
Applying the Open/Closed Principle with PHP 8 and PSR Best Practices: To make our PaymentProcessor class conform to the Open/Closed Principle, we can utilize PHP 8 features, such as interface improvements and union types, along with PSR best practices.
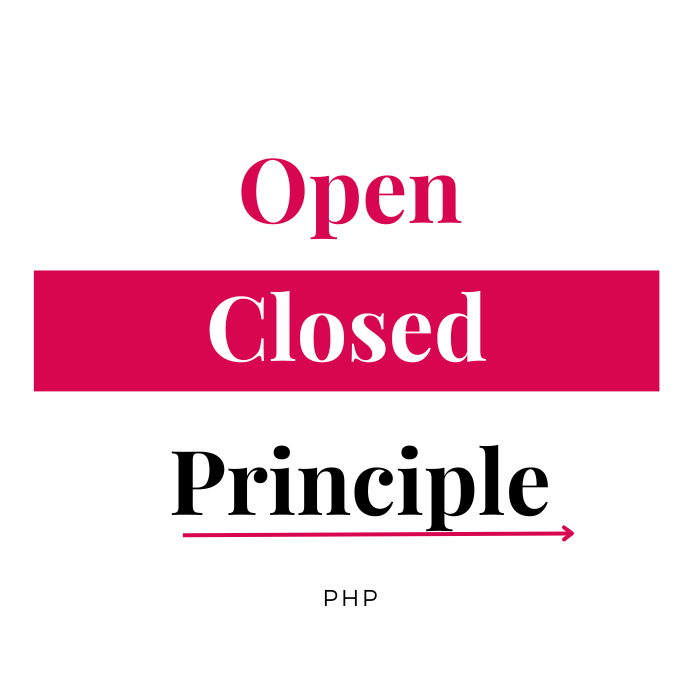
<?php
// PaymentMethodInterface.php
interface PaymentMethodInterface
{
/**
* Process a payment for the given amount.
*
* @param float $amount The amount to be paid.
* @return bool|string Returns true on successful payment or an error message on failure.
*/
public function processPayment(float $amount): bool|string;
}
<?php
// CreditCardPayment.php
class CreditCardPayment implements PaymentMethodInterface
{
public function processPayment(float $amount): bool|string
{
// Logic to process credit card payment
// Simulating a successful payment for the example
return true;
}
}
<?php
// PayPalPayment.php
class PayPalPayment implements PaymentMethodInterface
{
public function processPayment(float $amount): bool|string
{
// Logic to process PayPal payment
// Simulating a successful payment for the example
return true;
}
}
<?php
// PaymentProcessor.php
class PaymentProcessor
{
public function processPayment(PaymentMethodInterface $paymentMethod, float $amount): bool|string
{
return $paymentMethod->processPayment($amount);
}
}
With the PaymentProcessor
class and the CreditCardPayment
and PayPalPayment
classes in place, we can now use them as follows:
<?php
$paymentProcessor = new PaymentProcessor();
$creditCardPayment = new CreditCardPayment();
$paymentProcessor->processPayment($creditCardPayment, 100.00);
$paypalPayment = new PayPalPayment();
$paymentProcessor->processPayment($paypalPayment, 50.00);
Now, our PaymentProcessor class is open for extension through new payment methods but closed for modification. Adding a new payment method, such as ‘Stripe’, is as simple as creating a new class that implements the PaymentMethodInterface.
By embracing the Open/Closed Principle in PHP 8 and following PSR best practices, we create a flexible and maintainable codebase. Adhering to these principles not only ensures better scalability and code reusability but also promotes a more organized and developer-friendly environment. As your application evolves, you’ll appreciate the benefits of the Open/Closed Principle in keeping your codebase clean and efficient. Happy coding!